Hello World: Quick Start
The beginner's walkthrough to create and run a "Hello World" task in LOC.
-
To create a simplest data process with one generic logic and one aggregator logic. The data process would return a simple "Hello World" message.
-
Execute the data process as a task and view its execution result.
Data Process Design
Logic
Logic | Type | Name | Purpose |
---|---|---|---|
#1 | Generic | Hello World | Write string "Hello World!" into the session storage. |
#2 | Aggregator | Hello World Aggregator | Get the string "Hello World!" from session storage and finalise it in the task result. |
Task Payload
None required.
Task Result
{
"status": "ok",
"taskId": "...",
"message": "Hello World!"
}
Sign in LOC Studio
See: Signing In
Make sure you have an account for your LOC instance, open LOC Studio in the web browser and sign in.
Create Logic Entry Files
See: Create an Entry File
In order to create a data process (a data pipeline that can be run as a task), it requires at least one generic logic and only one aggregator logic. We will create two simple logic for this tutorial.
Create a Generic File
-
Go to Logic ➞ Logic Source and select Javascript repository, Typescript repository or C# repository depending on the language of your choice.
-
Right-click on
entry_files
and select New Folder to create a folder (for example, named using your own name). -
Right-click on the folder and select New Generic File.
-
Name the entry file as
hello-world.js
,hello-world.ts
orhello-world.cs
depending on the language of your choice. -
While still selecting the file, click the Edit icon on the top right.
-
Copy the following code (the language you've chosen) and paste into the editor to replace the template script, then click the Save icon.
-
Click the Cancel icon to close editing mode.
- JavaScript
- TypeScript
- C#
import { LoggingAgent, SessionStorageAgent } from "@fstnetwork/loc-logic-sdk";
/** @param {import('@fstnetwork/loc-logic-sdk').GenericContext} ctx */
export async function run(ctx) {
// log a message
LoggingAgent.info("Hello World!");
// write message to session storage
await SessionStorageAgent.putString("message", "Hello World!");
}
/**
* @param {import('@fstnetwork/loc-logic-sdk').GenericContext} ctx
* @param {import('@fstnetwork/loc-logic-sdk').RailwayError} error
*/
export async function handleError(ctx, error) {}
import {
GenericContext,
LoggingAgent,
RailwayError,
SessionStorageAgent,
} from "@fstnetwork/loc-logic-sdk";
export async function run(ctx: GenericContext) {
// log a message
LoggingAgent.info("Hello World!");
// write message to session storage
await SessionStorageAgent.putString("message", "Hello World!");
}
export async function handleError(ctx: GenericContext, error: RailwayError) {}
public static class Logic
{
public static async Task Run(Context ctx)
{
// log a message
await LoggingAgent.Info("Hello World!");
// write message to session storage
await SessionStorageAgent.Put(
"message",
StorageValue.FromString("Hello World!")
);
}
public static async Task HandleError(Context ctx, Exception error)
{
}
}
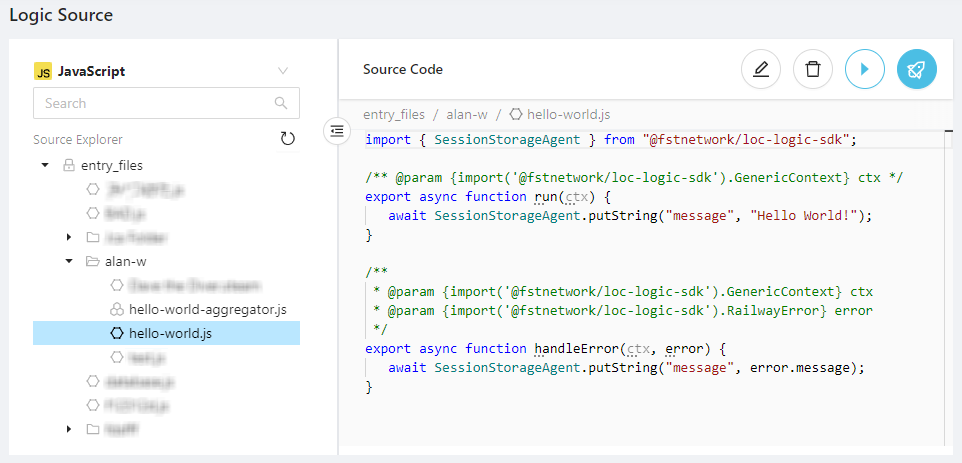
Create a Aggregator File
-
In the same manner as above, right-click on your folder and select New Folder to create a folder (for example, named using your own name).
-
Right-click on the folder and select New Generic File.
-
Name the entry file as
hello-world-aggregator.js
,hello-world-aggregator.ts
orhello-world-aggregator.cs
depending on the language of your choice. -
While still selecting the file, click the Edit icon on the top right.
-
Copy the following code (the language you've chosen) and paste into the editor to replace the template script, then click the Save icon.
-
Click the Cancel icon to close editing mode.
- JavaScript
- TypeScript
- C#
import { ResultAgent, SessionStorageAgent } from "@fstnetwork/loc-logic-sdk";
/** @param {import('@fstnetwork/loc-logic-sdk').AggregatorContext} ctx */
export async function run(ctx) {
// read message from session storage
const message = await SessionStorageAgent.get("message");
// finalise task result
ResultAgent.finalize({
status: "ok",
taskId: ctx.task.taskKey.taskId,
message: message,
});
}
/**
* @param {import('@fstnetwork/loc-logic-sdk').AggregatorContext} ctx
* @param {import('@fstnetwork/loc-logic-sdk').RailwayError} error
*/
export async function handleError(ctx, error) {
// finalise task result with error
ResultAgent.finalize({
status: "error",
taskId: ctx.task.taskKey.taskId,
message: error.message,
});
}
import {
AggregatorContext,
RailwayError,
ResultAgent,
SessionStorageAgent,
} from "@fstnetwork/loc-logic-sdk";
export async function run(ctx: AggregatorContext) {
// read message from session storage
const message: string = await SessionStorageAgent.get("message");
// finalise task result
ResultAgent.finalize({
status: "ok",
taskId: ctx.task.taskKey.taskId,
message: message,
});
}
export async function handleError(ctx: AggregatorContext, error: RailwayError) {
// finalise task result with error
ResultAgent.finalize({
status: "error",
taskId: ctx.task.taskKey.taskId,
message: error.message,
});
}
public static class Logic
{
public static async Task Run(Context ctx)
{
// read message from session storage
string? message = (await SessionStorageAgent.Get("message"))?.StringValue;
// read task metadata
var task = await ctx.GetTask();
// finalise task result
await ResultAgent.SetResult(
new Dictionary<string, object?>()
{
{ "status", "ok" },
{ "taskId", task.TaskKey.TaskIdString() },
{ "message", message }
}
);
}
public static async Task HandleError(Context ctx, Exception error)
{
// finalise task result with error
var task = await ctx.GetTask();
await ResultAgent.SetResult(
new Dictionary<string, object?>()
{
{ "status", "error" },
{ "taskId", task.TaskKey.TaskIdString() },
{ "message", error.Message }
}
);
}
}
Build Logic
The next step is to compile and deploy the logic using the source entry files.
Build Generic Logic
-
In the logic source, select
hello-world.*
and click the Build icon on the top right. -
In the build window, select Create new and enter the name Hello World.
-
Click Build.
Build Aggregator Logic
-
In the same manner of above, select
hello-world-aggregator.*
and click the Build icon on the top right. -
In the build window, select Create new and enter the name Hello World Aggregator.
-
Click Build.
Create Project and Scenario
Create a project (for example, <Your Name>'s' Project
) and a scenario (for example, Tutorial
) so that you can create data processes.
Create Data Process
See: Create Data Process
Now with the two logic, a project and a scenario created, we can create a data process to include these two logic.
-
In LOC Studio, go to Data Process ➞ Explorer.
-
Select a scenario and click Create Data Process on the top right.
-
Name the data process as
Hello World
or your name of choice. -
Click Add Generic Logic, select
hello-world.*
in the list and click Add. -
Click Add Aggregator Logic, select
hello-world-aggregator.*
in the list and click Add. -
Back in the data process creation window, click Create.
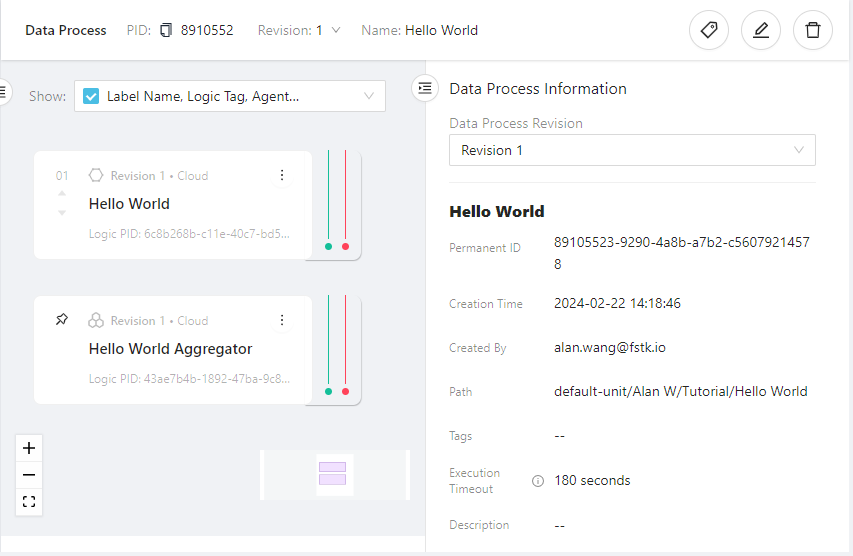
-
Have you built the logic and was it built successfully? If not sure, go to Cloud Logic to check if it exist, and go to Build History to check the build status.
-
Have you accidentally create and build the wrong type of logic (generic or aggregator)?
Run the Task
-
In the data process explorer, select the
Hello World
data process and click Execute Data Process on the top right. -
Select Execute as API Route Task.
-
Skip the payload and click Execute.
-
Wait for the task execution to finish.
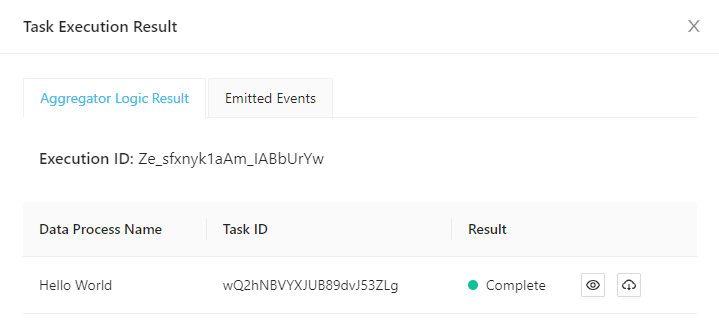
- Click the Preview icon under the Result column title to inspect the task result.
{
"message": "Hello World!",
"status": "ok",
"taskId": "wQ2hNBVYXJUB89dvJ53ZLg"
}
You can see the task has been successfully executed and has returned a JSON data containing the "Hello World" message.